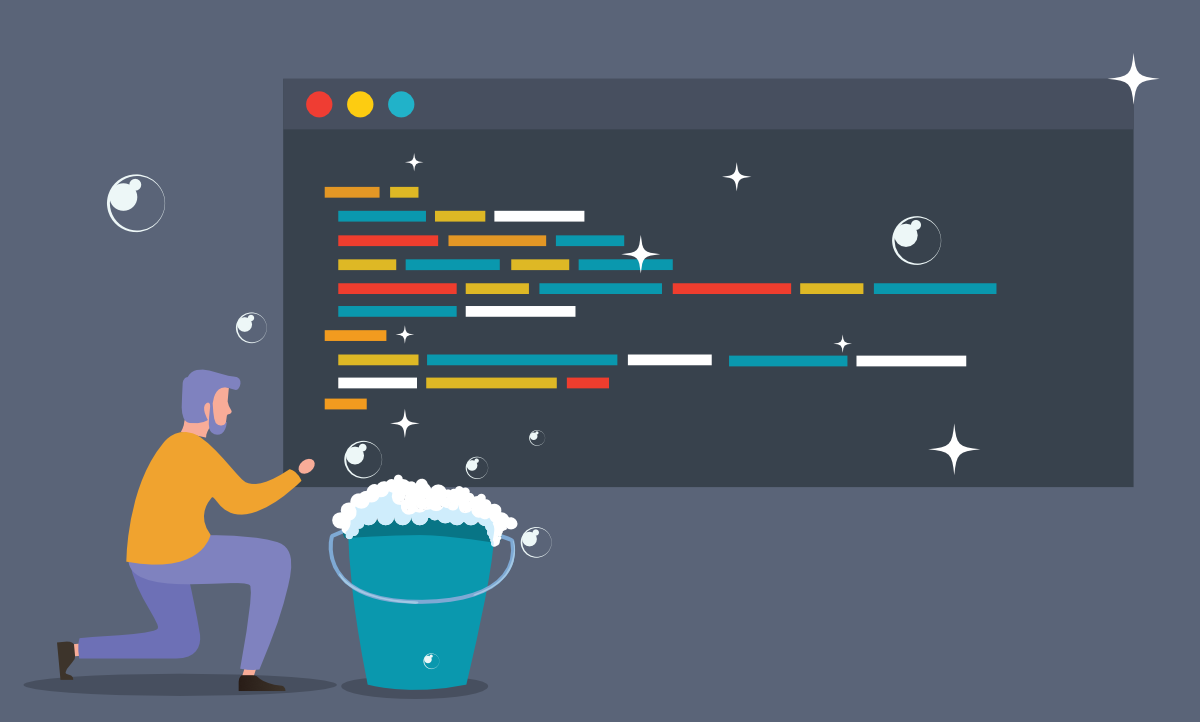
5 tips writing code in JavaScript as a Pro
When working with JavaScript, we deal a lot with conditionals, here are the 5 tips for you to write better / cleaner conditionals.
a year ago
#javascript
In this article, we'll explore the power of JavaScript one-liners and how they can improve the quality and readability of our code. One-liners refer to concise code snippets that accomplish tasks in a single line, streamlining our coding process.
Traditional Approach:
let numbers = [1, 2, 3, 4, 5];
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
One-liner:
let numbers = [1, 2, 3, 4, 5];
let sum = numbers.reduce((acc, num) => acc + num, 0);
Traditional Approach:
let numbers = [1, 2, 2, 3, 4, 4, 5];
let uniqueNumbers = [];
for (let i = 0; i < numbers.length; i++) {
if (!uniqueNumbers.includes(numbers[i])) {
uniqueNumbers.push(numbers[i]);
}
}
One-liner:
let numbers = [1, 2, 2, 3, 4, 4, 5];
let uniqueNumbers = [...new Set(numbers)];
Traditional Approach:
let a = 10;
let b = 20;
let temp = a;
a = b;
b = temp;
One-liner:
[b, a] = [a, b];
Traditional Approach:
let str = 'hello';
let reversedStr = '';
for (let i = str.length - 1; i >= 0; i--) {
reversedStr += str[i];
}
One-liner:
let str = 'hello';
let reversedStr = str.split('').reverse().join('');
Traditional Approach:
let numbers = [1, 2, 2, 3, 4, 4, 5];
let count = {};
for (let i = 0; i < numbers.length; i++) {
if (count[numbers[i]]) {
count[numbers[i]]++; }
else {
count[numbers[i]] = 1;
}
}
One-liner:
let numbers = [1, 2, 2, 3, 4, 4, 5]; let count = numbers.reduce((acc, num) => { acc[num] = (acc[num] || 0) + 1; return acc; }, {});
Traditional Approach:
let myObject = { name: 'Alice', age: 30 };
let hasKey = false;
for (let key in myObject) {
if (key === 'age') {
hasKey = true;
break;
}
}
One-liner:
let myObject = { name: 'Alice', age: 30 };
let hasKey = 'age' in myObject;
Traditional Approach:
let array1 = [1, 2, 3, 4];
let array2 = [3, 4, 5, 6];
let intersection = [];
for (let i = 0; i < array1.length; i++) {
for (let j = 0; j < array2.length; j++) {
if (array1[i] === array2[j]) {
intersection.push(array1[i]); break;
}
}
}
These one-liners simplify common tasks and make your code more concise and readable. Incorporating them into your coding practices can lead to more efficient development. Keep these tricks in mind for your day-to-day coding adventures!